Token based security
Using JWT tokens to determine if a user can access the stream or not
THEOlive offers the option to enable JWT token security on channel alias level. This can be interesting if you only want valid users to access your stream.
In this document, we will
- explain how it works
- how to enable/disable it via the console and API
- how to configure your THEOlive player to pass the mandatory headers
How it works
When enabling token security on a channel alias, we expect you to share the shared (private) key in case of HS256/HS512 or the public key in case of RS256/RS512 encryption. This will be used on CDN level to determine if a request (with a Bearer token passed in the Authorization
header) is valid or not. It's up to you to configure this header correctly through our THEOlive npm player. Later in this document we will explain how.
In the bearer token that gets sent to us, we expect the following properties to be available:
exp
: date in epoch format until which the JWT token is validnbf
: optional date in epoch format. Stands for "not before" and acts as a "start date" of the JWT to be valid.
When not passing a bearer token for a secured channel, the request will be rejected.
Enable or disable token security for an alias
Please refer to the Enable token security for alias and Disable token security for alias API endpoints to manage the token security settings for an alias.
When enabling for the first time, you have to pass the key
property in the body. If you disable token security later on, and make it active again, you can omit this property if it should stay the same.
If you're using the THEOlive management console, you can navigate to a player details page and select the alias you want to enable/disable token security for. When enabling, please pass the correct shared or public key to use. Don't forget to confirm your changes by hitting the save button!
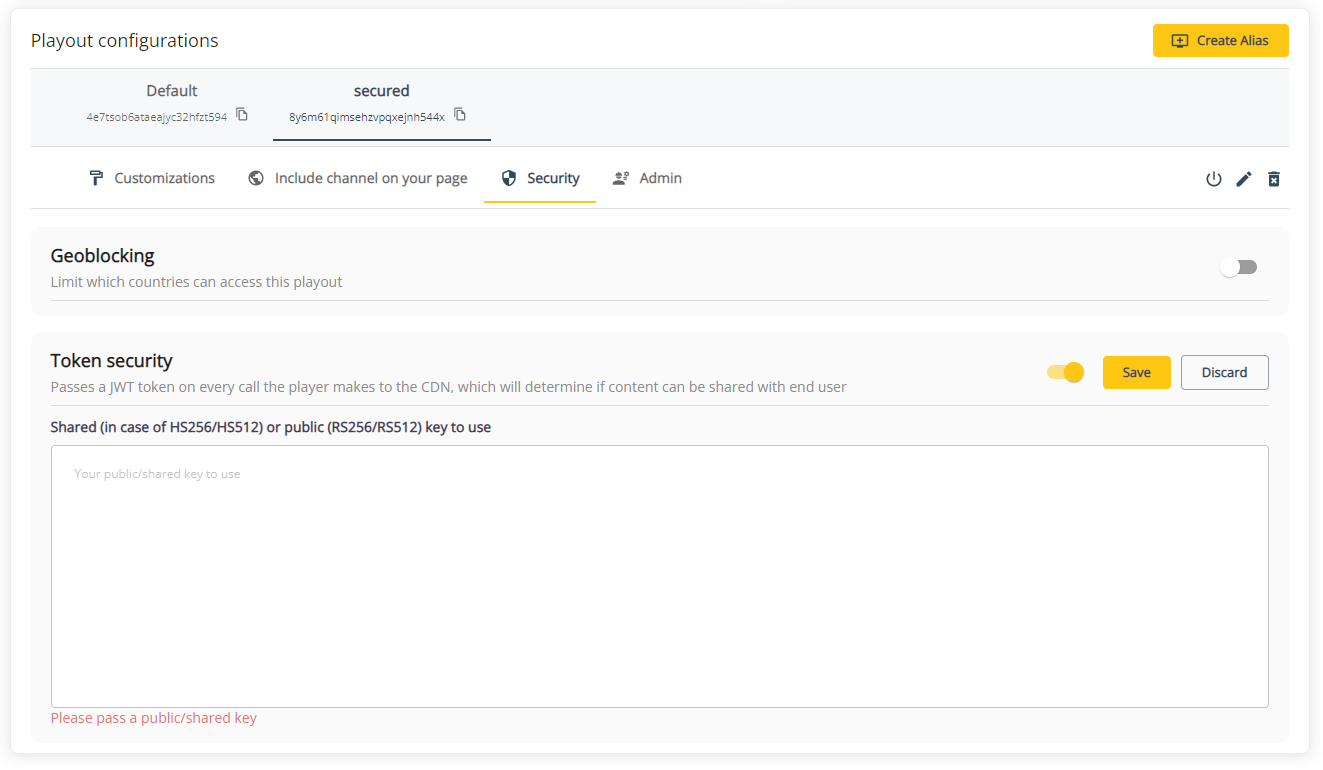
Managing token security in the console
Passing headers with the THEOlive npm player
In order to integrate token based security in your application, you have to make use of the THEOlive npm player. Passing headers isn't possible when using the embed player!
1. Create a header provider
First thing to do is to create a header provider function. In this function you add the Authorization
header which contains a bearer token generated by you. This token should at least contain a exp
property, and an optional nbf
property. You can add as many other properties to it as you want, but THEOlive doesn't do anything with them.
const headerProvider = (url) => {
return new Headers({
'Authorization': 'Bearer <token-generated-by-you>'
});
}
2. Pass the header provider to the THEOlive player
Once you've created the header provider, you have to pass it to the THEOlive player instance. This can be done in the following way:
const player = new THEOLive.Player(document.getElementById('player'));
player.addHeaderProvider(headerProvider);
The player will now include the header in every request that gets made towards the CDN to grab content. Passing no or an invalid token will result in an unauthorized error and prevent users from watching the stream.
Updated 6 months ago